Simple forms development with React.js and Formio.js
Building forms always takes a considerable amount of time and effort to make it work properly end to end. Why? Because it usually needs to have a proper validation in some fields, uses different input types, it needs to be easy to use (UX) and have a decent design (UI).
There are many things to take care of, that is why in this article we will learn how to use Formio.js
This library is a plain JavaScript form renderer and SDK for Form.io. This allows you to render the JSON schema forms produced by Form.io and render those within your application using plain JavaScript, as well as provides an interface SDK to communicate to the Form.io API. The benefits of this library includes:
- Plain JavaScript implementation using ES6 and Modern practices (no jQuery, Angular, React, or any other framework dependency)
- Renders a JSON schema as a webform and hooks up that form to the Form.io API’s
- Nested components, layouts, Date/Time, Select, Input Masks, and many more included features
- Full JavaScript API SDK library on top of Form.io
- Provides supports to integrate with React, Vue, Angular, and more libraries.
Note: For this article, I’m using a basic React.js app, that is why we are going to use the alternative of the library to render Formio as a React.js component.
Enough talk, let’s show some code:
1) We create a react app for our example:
npx create-react-app my-app
2) Once we have the app, we install Formio.js for React:
cd my-app npm install react-formio --save
For this example, we use the src alternative, which allows using a JSON file locally that contains the form schema.
3) We add a formData.json file on same folder where is App.js
4) The easiest way to get the form we need, is using the form builder that Formio provides.
On this page, you can drag and drop any component, set configurations, or change anything using UI. On the left side there are many options to choose:
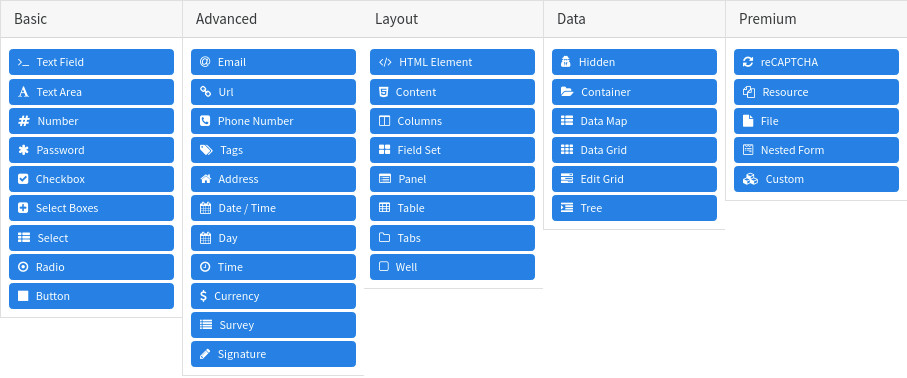
Once you are done, on the right side of the page you will see the JSON schema. This schema is what we need to add to our JSON file.
Copy the entire JSON schema and paste it into our formData.json, then remove the “settings” section (this part is not needed). On my example, I’m using this schema:
{ "display": "form", "components": [ { "label": "First Name", "tableView": true, "key": "name", "type": "textfield", "input": true }, { "label": "Last Name", "tableView": true, "key": "lastName", "type": "textfield", "input": true }, { "label": "Plan", "widget": "choicesjs", "tableView": true, "data": { "values": [ { "label": "Free", "value": "free" }, { "label": "Paid", "value": "paid" } ] }, "selectThreshold": 0.3, "key": "select", "type": "select", "indexeddb": { "filter": {} }, "input": true, "defaultValue": "free" }, { "label": "Phone Number", "tableView": true, "key": "phoneNumber", "type": "phoneNumber", "input": true }, { "label": "Email", "tableView": true, "key": "email", "type": "email", "input": true }, { "type": "button", "label": "Submit", "key": "submit", "disableOnInvalid": true, "input": true, "tableView": false } ] }
5) We add a new MySimpleForm.js file for our form component that will contain the following:
import React from 'react'; import {Form} from 'react-formio'; import formData from './formData.json'; import 'formiojs/dist/formio.builder.min.css' class MySimpleForm extends React.Component { handleSubmit(data) { console.log(data); } render() { return ( <div style={{maxWidth: '400px', margin: '50px'}}> <Form form={formData} onSubmit={this.handleSubmit}/> </div> ) } } export default MySimpleForm
6) We add the import statements on the App.js to invoke our MySimpleForm.js form component
import MySimpleForm from './MySimpleForm';
and replace the content inside the div element that wraps everything on App with:
<MySimpleForm/>
The App.js should look like this:
import React from 'react'; import logo from './logo.svg'; import MySimpleForm from './MySimpleForm'; import 'bootstrap/dist/css/bootstrap.min.css' import './App.css'; function App() { return ( <div className="App"> <MySimpleForm/> </div> ); } export default App;
7) Now that we have all in place, let’s run the app:
npm run start
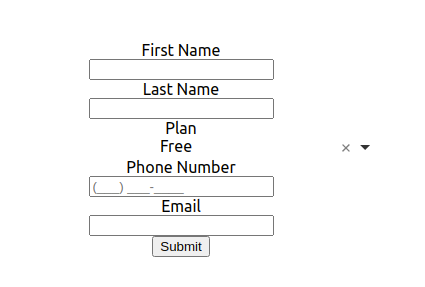
You might notice that the form on the page has pretty much the same structure you defined at the form builder, but it doesn’t look very nice, that is because Formio.js responsibility is to build the form, add validations, and other behaviors. It’s up to you whether to use a CSS framework or add your own custom style.
8) Extra: Testing how if we install Bootstrap
npm install bootstrap --save
and add the styles to MySimpleForm.js:
import 'bootstrap/dist/css/bootstrap.min.css'
It should look like this:
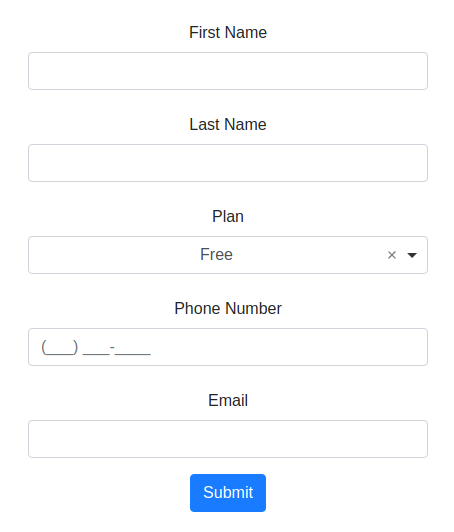
In case you are wondering how you can prefill the fields (to use it in an edit form), you can use the submission attribute that can handle an object with data for each field.
You can find more information about this cool library at:
- Official documentation: https://github.com/formio/formio.js/wiki
- React library that renders Formio: https://github.com/formio/react-formio
- Many examples: https://formio.github.io/formio.js/app/examples/
It doesn’t work with select field, when we select, it can not save the data